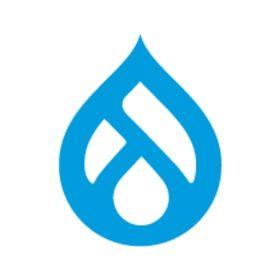
By Eagle11 on
I have a module that overrides the alter_hook form. The form created is a form created by the core form tool in Drupal 8. When I try to modify one of the fields, I cannot submit the form, but get an error:
Fatal error: Cannot unset string offsets in .../core/lib/Drupal/Core/Field/WidgetBase.php on line 356
Comments
Probably something you did
Probably something you did wrong in your code.
That's a safe assumption
That's a safe assumption, I guess. It's a very simple .module file in a custom module, overwriting a single field in the form. Below is the entire code.
I think that this:
I think that this:
Should be this:
I just tried your fix,
I just tried your fix, refreshed the caches, and still no.
After experimenting quite a lot, I found that if I remove this part of the array,
'#type' => 'textfield'
I can submit the form successfully. But if I add that in, it fails. That is the only key value pair that causes the form to not submit. Of course, the field doesn't actually render on the page without that pair though.
What does this dump to the
What does this dump to the page?
This is the result:
This is the result:
As you can see, the element
As you can see, the element is
[#type] => container
, and you are changing it to a textfield. This is confusing the submit handler that is expecting some child of the container that you haven't set.Rather than re-declaring the field altogether, you should be altering the values you want to change. Examine that dump that you just posted, and change the values you want to be different.
I tried that. At least I
I tried that. At least I tried it the only way I know how, which may be wrong. So if I do this instead:
Which leaves off the textfield and the required, the field does not render. Am I correct in how I'm overriding this field?
You are re-declaring the
You are re-declaring the field (which entirely overrides the existing field). You need to alter the elements that you want to change.
What specifically are you trying to do with this code?
My main objective is to add
My main objective is to add the placeholder text and the prefix and suffix.
Well it looks like the actual
Well it looks like the actual element you want to alter is
$form['field_first_name']['widget'][0]
, so you'll want to make your changes to this.Ex:
That was it! Not very
That was it! Not very intuitive to me, but hey, it works! Thanks so much for sticking with me!
No problem :)
No problem :)
Here's a little more explanation. Imagine we have this array:
Let's imagine you want to change the title. If you do this:
Now your array dump will be this:
As you can see, there is only one element in the array now, because the array was re-declared (overwritten).
To change the title, you don't change $element, you change $element['#title']:
Now the dump will be:
As you can see, by only overwriting $element['#title'], it leaves the other field(s) intact, instead of wiping it out.
In hook_form_alter(), you should almost always be altering elements of array, not re-declaring the array.
Yes, that makes sense now.
Yes, that makes sense now. You make a good teacher. :)
Same Error while submitting the contact form.
Error Message::
Fatal error: Cannot unset string offsets in .../core/lib/Drupal/Core/Field/WidgetBase.php on line 356
Contact form subject field is altered with 'select list' in custom module.
When I am submitting the form, the same error is generating.
Code::
if($form_id=="contact_message_contact_us_form"){
$form['subject'] = [
'#type' => 'select',
'#title' => 'Subject',
'#options' => [
'0' => 'yes',
'1' => 'No'
],
];
}