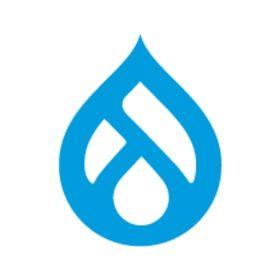
By Dakanji on
Trying to hook into Drupal 7 from an external php file by using various variants of ...
function getDrupalHTML() {
define('DRUPAL_ROOT', getcwd());
require_once DRUPAL_ROOT . '/includes/bootstrap.inc';
drupal_bootstrap(DRUPAL_BOOTSTRAP_FULL);
ob_start();
menu_execute_active_handler();
$contents = ob_get_clean();
return $contents;
}
However, it seems D7 just spits out the HTML to the browser on the menu_execute_active_handler()
call.
Any ideas how to get the html output into my variable?
Thanks
Comments
Tracking the Drupal flow gives answer
Tracking the Drupal flow line by line and function by function reveals the culprit to be ob_flush in the drupal_page_footer function
Using the following instead of ob_flush would make the application a bit more friendly
Just curious, could you not
Just curious, could you not just use hook_exit(), get the data then stop the script execution using drupal_exit(), that way ob_flush is never called.
Actually, I think you can
Actually, I think you can skip all that and just do the following:
Thanks but drupal_page_footer
Thanks but drupal_page_footer is called downstream of drupal_deliver_html_page so the expected outcome is the same.
Will look into this later
For now, I have just implemented my versions of the relevant drupal functions.
Now works fine but this may be more elegant.
Finally solved
Finally solved by adding a delivery callback to my module_menu function and handling things there to bypass the problem function.