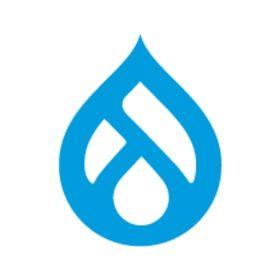
Problem/Motivation
I have begun using Entity Print to print out PDF copies of receipts for Drupal Commerce orders. I have hooked into the email system so that when the emailed receipt goes out to users, a copy of the receipt is added as a PDF. I have noticed that the receipt email has the shipping address, but the PDF copy created by entity print does not include the shipping address. I have checked the templates and made them identical, but the shipping address isn't getting passed through entity print. Billing address does appear, however, on both the email receipt and the PDF receipt.
Example code
/**
* Implements hook_mail_alter
*/
function mymodule_mail_alter(&$message) {
if ($message['id'] === 'commerce_order_receipt') {
$order_id = $message['params']['order']->id();
$order = Order::load($order_id);
$order_number = $order->get('order_number')->value;
$filename = 'Order' . $order_number . '-' . date('m-d-Y') . '.pdf';
mymodule_entity_print_saver($order_id, $filename);
$message['params']['attachments'][] = [
'filecontent' => file_get_contents('temporary://' . $filename),
'filename' => $filename,
'filemime' => 'application/pdf',
];
if ($order->bundle() === 'repair_services') {
$message['to'] = $order->field_email->value;
}
}
}
/**
* Save order as PDF.
*/
function mymodule_entity_print_saver($order_id, $filename, $scheme = 'temporary') {
$pb = new PrintBuilder(
\Drupal::service('entity_print.renderer_factory'),
\Drupal::service('event_dispatcher'),
\Drupal::service('string_translation')
);
$order = Order::load($order_id);
$print_engine = \Drupal::service('plugin.manager.entity_print.print_engine')->createSelectedInstance('pdf');
$path = $pb->savePrintable(
[$order],
$print_engine,
$scheme,
$filename
);
$file = File::create([
'uri' => $path,
'uid' => 1,
'status' => FileInterface::STATUS_PERMANENT,
]);
$file->save();
return $file;
}
Proposed resolution
My guess is that this has to do with the fact that commerce shipping is a separate module and the shipping profile isn't searched for like the billing profile is. Not sure exactly how to fix it.
Comments