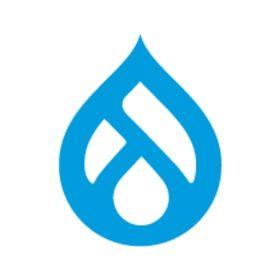
Problem/Motivation
in attributesToOptions
function there is some code to transform attributes names to camelcase, instead we can use dataset directly as it is supported everywhere we support.
https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/dataset
Steps to reproduce
Proposed resolution
Remaining tasks
User interface changes
API changes
Data model changes
Issue fork a11y_autocomplete-3229110
Show commands
Start within a Git clone of the project using the version control instructions.
Or, if you do not have SSH keys set up on git.drupalcode.org:
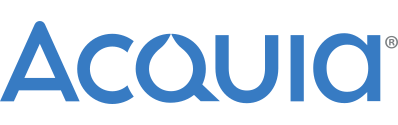
Comments
Comment #2
nod_Comment #3
nod_for testing you can use
in the browser console run this code and then execute
attributesToOptions()
to see the result of the transformation of the data attributes into an option object. Or you can add that code to a html file and open it might be easier than using the console all the time.Comment #4
yash.rode CreditAttribution: yash.rode at Acquia commentedcheck data type of dataset and d, currently it is var can be changed to let if needed.
Comment #6
nod_There are tests to check the behavior of this function so please update the merge request and make sure the tests are passing :)
Comment #7
yash.rode CreditAttribution: yash.rode at Acquia commentedComment #8
nod_a few details and it'll be ok to merge.
Comment #9
yash.rode CreditAttribution: yash.rode at Acquia commentedComment #10
nod_Need to change do the let -> const change in the for loop also
Comment #11
yash.rode CreditAttribution: yash.rode at Acquia commentedComment #12
yash.rode CreditAttribution: yash.rode at Acquia commentedComment #13
nod_all good :)
Main change is that using dataset allow us to get rid of this piece of code:
because with dataset this is done automatically by the browser.
Comment #15
nod_