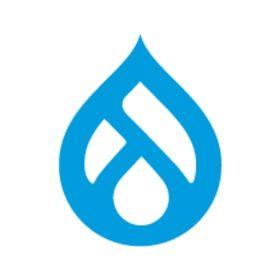
i tried adding a form field for doing search on the page that returns the results. in the same fashion as i mentioned here (#2369289: is it possible to submit a form with a carriage return?). but this time i added a button for submit (since CR simply crashes the app). this was more odd behavior. first off i need the form to submit and then return to itself to display results and then have the field available to enter a new search term. so i did my trick of having a dummy page to submit to.
this works fine. but only the first time. every time after that that i resubmit, even though my _submit function is hit; the form_state[values] are empty.
i'll post slightly cleaned up code. btw.. maybe just my inexperience with js but i didnt see how to pass search terms through function chain. at first i thought as part of url, but as far as i can tell i can't do a menu with a % and still have that list in menu block (i.e. with item['search/%'] putting "search/%" as path in drupalgap.settings.menus['main_menu'] causes an error. so only way i could figure to do this was with a "global" var. this might be the root of my issue?
var global_search_terms; // didnt know how else to pass search terms
function placemarks_search_form(form, form_state) {
form.elements.search_terms = {
title:'search',
type:'textfield',
};
form.elements['submit'] = {
type: 'submit',
value: 'Search'
};
return form;
}
function placemarks_search_form_submit(form, form_state) {
var termstr = form_state['values']['search_terms'];
global_search_terms = termstr.trim().split(/\s+/).join('+');
if (drupalgap_get_page_id() == 'placemarks_search') {
drupalgap_goto('placemarks_search_dummy', {reloadPage:true});
}
else {
drupalgap_goto('placemarks_search', {reloadPage:true});
}
}
function placemarks_search_page() {
try {
return {
'search_form': {
'markup': '<div class="search-page-search-form"></div>'
},
'placemarks_search_results':{
'theme':'jqm_item_list',
'title':'Results',
'items':[],
'attributes':{'class':'placemarks_search_results'},
}
};
}
catch (error) { console.log('placemarks_search_page - ' + error); }
}
function placemarks_search_pageshow() {
try {
dpm(global_search_terms);
$('.search-page-search-form').html(drupalgap_get_form('placemarks_search_form'));
var path_to_view = 'placemarks/search/json/' + global_search_terms;
if (global_search_terms.length > 0) {
views_datasource_get_view_result(path_to_view, {
'success':function(data){
if (data.nodes.length > 0) {
var items = [];
$.each(data.nodes, function(index, object){
var node = object.node;
items.push(l(node.name, 'node/' + node.nid));
});
drupalgap_item_list_populate(".placemarks_search_results", items);
}
//global_search_terms = '';
}
});
}
}
catch (error) {console.log('placemarks_search_pageshow - ' + error);}
}
as i mentioned, this works, but only the first time. after submitting a search term and getting back to that page with results; i do get the field displayed above the results. but entering another term and searching only returns empty results page. i can see that subsequent calls to _submit function have form_state['values']['search_terms'] empty.
another noteworthy point in that i had to use a class rather than an id to push results to due to my dummy page idea. my guess is that results need to push to both pages since technically i think there are really 2 and they can't have the same id for 2 divs and the one the results get pushed to is not the one on the page i redirect to (not sure that makes sense outside my head).
Comments
Comment #1
tyler.frankenstein CreditAttribution: tyler.frankenstein commentedIn cases were I want a very dynamic page (i.e. reloading assets into it without navigating away), I may skip the DrupalGap Forms API and just use widgets and theme_* functions to build a "fake form". Then just attach onclick handlers and various other attributes to handle user interataction, and then fetch new results and inject them into the page.
The rest of this is too much for me right now, I will have to check it out another time.
Comment #2
liquidcms CreditAttribution: liquidcms commentedyes, redid this as a std form page and it works well. still with the dummy page trick to submit and return to itself with results:
http://screencast.com/t/V3qcQ6T8t7l
http://screencast.com/t/VJ0xYH6dJ
http://screencast.com/t/4G2ecFrc6XvX
http://screencast.com/t/IU9df8cbY
only thing i havent sorted out is how to mix the idea of a drupal_get_form page callback with the jqm_item_list array returned with the pageshow filling in the values. i don't get what that array is. is it possible to render this and place it in a form element so i can merge a form callback with drupalgap_item_list_populate()?
Comment #3
tyler.frankenstein CreditAttribution: tyler.frankenstein commentedI'm not sure I entirely understand here...
What "array" you are referring to? I'm guessing the "items" array needed by jqm_item_list and item_list. Essentially it is equivalent to Drupal's "items" array when using theme('item_list', ...);
In DrupalGap, you pass an items array full of rendered html strings, and it'll inject each of those items inside an ul/li or ol/li item.
You may have to use
$('#my_item_list').trigger('create');
to force jQM to redraw the items in a nice way, but jqm_item_list should take care of that automatically for you.Comment #4
liquidcms CreditAttribution: liquidcms commentedthe examples for using jqm_item_list simply return the "render" array in the page callback, like such:
and then use pageshow call back to populate:
but, as suggested i am now using drupal_get_form as my page call back and passing it a form definition. so i need to return a form structure. so to push my item list to my form i currently have this element in my form:
so question becomes, how do i get this:
into my form?
Comment #5
tyler.frankenstein CreditAttribution: tyler.frankenstein commentedTry this:
Comment #6
liquidcms CreditAttribution: liquidcms commentedyup, that works. :)
thanks. again.
Comment #7
aegirone CreditAttribution: aegirone commentedI have the same issue when i build the search function for our app, only first time it works, after it it does not work.
Comment #8
liquidcms CreditAttribution: liquidcms commentedi posted here: https://github.com/signalpoint/DrupalGap/issues/254#issuecomment-61556030