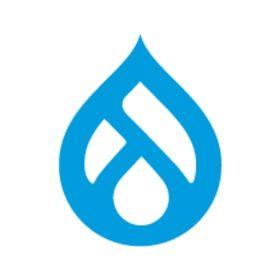
Currently the recruiter role can see a link at the bottom of a job node that says "Apply for this job", even though they do not have permission to apply for jobs.
I can't see why a recruiter would want to apply to their own jobs.
Clicking the link says "You are not authorized to apply for jobs".
Is there a way of hiding this link from a recruiter by adding another else statement in the hook_link?
function job_link($type, $node = NULL, $teaser = FALSE) {
global $user;
$links = array();
if ($type == 'node') {
// We are viewing a node
if (variable_get(JOB_NODE_TYPE . $node->type, 0)) {
// Node type is enabled for job apply
if (!$user->uid) {
// User is not logged in
$links['job_apply'] = array(
'title' => t('Please login or register to apply'),
'href' => 'user/login',
);
}
else {
// User is logged in
if (_job_check($node->nid, $user->uid)) {
// User has applied for this job
$links['job_apply'] = array(
'title' => t('You applied for this job'),
);
return $links;
}
else {
// User has not applied for this job
$links['job_apply'] = array(
'title' => t('Apply for this job'),
'href' => "job/apply/$node->nid",
);
Comment | File | Size | Author |
---|---|---|---|
#11 | apply_fixes.patch | 3.09 KB | mandclu |
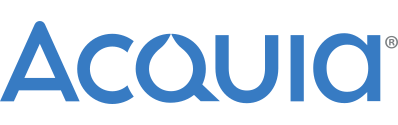
Comments
Comment #1
mandclu CreditAttribution: mandclu commentedIt's an interesting question. My first thought would be that it needs a user_access call around the contents of the last "else" condition.
I suppose the larger question is if there should be any visual cue to someone who is logged in but doesn't have the permission to apply.
You could also argue that the current logic assumes that only authenticated users can apply. That might lead one to conclude the user_access call should come before the user id check, and enclose that whole structure.
Comment #2
Peng.Pif CreditAttribution: Peng.Pif commentedI don't think a recruiter should be able to see the link. What benefit would it be to them?
I can see why it is in the module though. A job seeker may be logged in, but does not have permission to apply until a subscription is purchased etc. You may also need to suspend a job seekers account, due not receiving payment etc. In these 2 instances they will then receive the "You are not authorised to apply" message.
I have tried, but with no success to remove the link from view of a recruiter:
Thanks to WorldFallz for providing that snippet. Can anyone help out with this?
Thanks.
Comment #3
mandclu CreditAttribution: mandclu commentedI think we should be careful about writing this to be too specific about your particular needs. A recruiter won't need to apply for her own jobs, but might want to apply for a job by another recruiter. Also, testing for roles instead of permissions seems very un-Drupal.
I think we should take the time here to map out the use cases, and that will be the coding much, much easier.
Off the top of my head:
Someone who has permission to apply and isn't the person who posted the job:
Show the "Apply" link
An anonymous user who can't apply:
Show the "Login or Register" link
(sidebar: this implies that authenticated users have access to apply)
All others:
Show nothing?
Comment #4
Peng.Pif CreditAttribution: Peng.Pif commentedYes, you are right, I did not look at the bigger picture. A recruiter may need to apply for jobs. An example case would be:
A Job has been posted by Recruitment company 1. Recruitment comapny's 2 and 3 etc are applying for the Job on behalf of candidates (job seekers) who have registered with them. This is where having the choice of selecting from different resumes will come into play. Recruiters will need to be able to apply for this reason.
I agree with 1 (authenticated) and 2 (anonymous) you mentioned.
The main thing here is that you should not be able to see the link to apply on job nodes you created.
In regards to all others, can you explain what types of roles these would be please?
Thanks.
Comment #5
mandclu CreditAttribution: mandclu commentedThe "others" would be authenticated users who do not have permission to apply. To cite an example you mentioned earlier, it might be a user who hasn't yet paid for the access to apply. This one will be difficult to anticipate, as the best solution will probably vary widely based on how the job board is being set up. I suppose the Cadillac solution would be to provide a settings field that would allow an administrator to specify the message that would be shown to such users.
Comment #6
Peng.Pif CreditAttribution: Peng.Pif commentedFor the "others", yes it seems tricky to anticipate all possible use cases. Having a tiered level of job seekers could be very complex. A customisable message would then be the best solution. 'Please contact Admin for information on how to apply', as an example.
'Please login / register to apply' leads to the registration page, the customized link would lead to a contact form?.
Perhaps even lead to a page which could contain user inserted information (subsription details etc) which has a link to a contact form.
Comment #7
Peng.Pif CreditAttribution: Peng.Pif commentedHi, here is a snippet that hides the "Apply for this Job" from the Recruiter.
This will need to be modified to hide it from the view of the person who has posted the Job, as they would not want to apply for their own Job. But another Recruiter may want to apply for the Job, so it should be visible to that role.
Comment #8
mandclu CreditAttribution: mandclu commentedThat snippet has a couple of problems. First, it's dependant on a role called 'recruiter', which is a problem. Ideally it should be testing for the permission to apply, using a test like user_access(JOB_PERM_APPLY) instead.
Also, I thought we established in #4 that recruiter might need to be able to apply for jobs in some circumstances, so the business logic is off anyway.
I would suggest that the testing for permission to apply for a particular node be spun out to a separate helper function, something like
That way, the same logic can be used for deciding whether or not to show the apply link, as well as for determining access to the application menu callback.
Comment #9
mandclu CreditAttribution: mandclu commentedBased on that helper function, you could have the job_link function look something like:
Comment #10
Peng.Pif CreditAttribution: Peng.Pif commentedMy bad surge, yes we did establish in #4 that a Recruiter should be able to apply. I just posted the snippet as I thought it could be usefull to edit / change to do what we had discussed.
What you have posted makes much more sense and you also mentioned above that testing for roles instead of permissions is not the drupal way of doing things.
Will you roll a patch?
Thanks.
Comment #11
mandclu CreditAttribution: mandclu commentedOK, the attached patch implements the code above, as well as a tweak to use the permission function for the job_apply function. That latter function could probably use a little more attention too (some of its checks are now redundant) but this patch addresses the issue at hand.
Please test and let me know how this works.
Comment #12
Peng.Pif CreditAttribution: Peng.Pif commentedJust tested and this works perfect.
The link to apply is hidden from the node creator, but visible to all other authenticated users.
'Please login or register to apply' link is visible to anonymous users.
Comment #13
mandclu CreditAttribution: mandclu commented